Let’s GO with IPinfo! Starter guide on using IPinfo services on Go.
First, let’s get ourselves that IPinfo Token
Let’s get started with the simple thing and sign up for IPinfo’s services. You get 50,000 IP geolocation requests/month on the free tier alone. The process is simple:
- Signup by visiting this link: https://www.ipinfo.io/signup
- Go to your dashboard and copy your account token or, visit: https://ipinfo.io/account/token
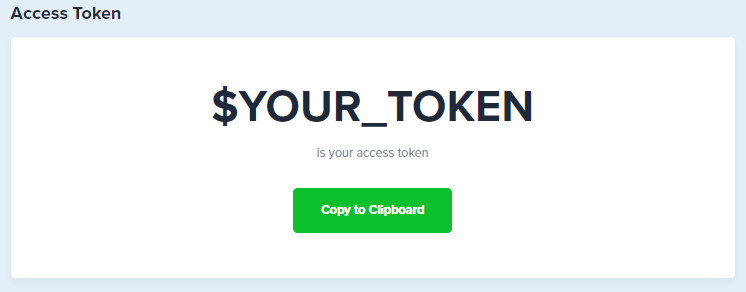
And now that you are good to go.
Setting up the Go programming environment
Open your terminal in your desired folder first. To use the IPinfo’s Go Package, first initialize a Go module with the go mod init
command:
go mod init ipinfo_example
Then get the IPinfo package with the go get
command:
go get github.com/ipinfo/go/v2/ipinfo
Now you are ready to get started using it.
Getting started
Let’s start with the initial setup, we need to do the following:
- Import the IPinfo package.
- Use your token in the
ipinfo.NewClient
function to create theclient
handler. - Get your IP related information using the
client.GetIPinfo
function. - Print out the information.
To import the package, add it to your import statement:
"github.com/ipinfo/go/v2/ipinfo"
Then, inside the main function, declare the token variable with your access token that you copied from your IPinfo account dashboard. Then pass it to the ipinfo.NewClient
function and to create the client
API handler.
const token = "$YOUR_TOKEN"
client := ipinfo.NewClient(nil, nil, token)
Notice the two nil
parameters in the NewClient
. They are used to declare the httpClient
and cache
operations, respectively. But for standard operation, you can set them as nil
.
Then, using the client
handler’s GetIPInfo
function, you can query IP geolocation and other information from an IP address. We are going to use the net.ParseIP
to parse the IP address from string type to IP address type before passing it to the client.GetIPInfo
. Then, after a catch error statement, we can print out the response.
// replace "8.8.8.8" with the IP address you would like to lookup
info, err := client.GetIPInfo(net.ParseIP("8.8.8.8"))
// catch error statement
if err != nil {
log.Fatal(err)
}
// Finally outputting the response
fmt.Println(info)
Boilerplate Code
All in all, your code should look something like this:
package main
import (
"fmt"
"log"
"net"
"github.com/ipinfo/go/v2/ipinfo" // importing the IPinfo package
)
func main() {
// make sure to add your token here
const token = "YOUR_TOKEN"
// initialize the client like so:
client := ipinfo.NewClient(nil, nil, token)
// Get the info with the client handler we just created
// replace the "8.8.8.8" with the IP address you want to lookup
info, err := client.GetIPInfo(net.ParseIP("8.8.8.8"))
// catch error statement
if err != nil {
log.Fatal(err)
}
// Finally outputting the
fmt.Println(info)
}

After that, save the file and in the terminal, you can go run
your program.
go run main.go
or, you can build an executable with go build
command:
go build main.go
main.exe
The output would be something like this:
{8.8.8.8 dns.google false true Mountain View California US United States false 37.4056,-122.0775 AS15169 Google LLC 94043 America/Los_Angeles <nil> <nil> <nil> <nil> <nil> <nil>}
Available Methods
If you are confused by what you are seeing, don’t be. Your IPinfo Go package uses structs
to declare the values. You can find more information about this in our GitHub Repo. Essentially, you need to call specific methods (a struct pointer to be exact) on each item to get the respective field.
Reference table on the struct
field names:
Method | Type | Usage | Example Output |
---|---|---|---|
IP | net.IP | info.IP | 69.205.168.250 |
Hostname | string | info.Hostname | 069-205-168-250.inf.spectrum.com |
Bogon | bool | info.Bogon | false |
Anycast | bool | info.Anycast | false |
City | string | info.City | Southfield |
Region | string | info.Region | Michigan |
Country | string | info.Country | US |
CountryName | string | info.CountryName | United States |
IsEU | bool | info.IsEU | false |
Location | string | info.Location | 42.4638,-83.2255 |
Org | string | info.Org | AS12129 123.Net, Inc. |
Postal | string | info.Postal | 48075 |
Timezone | string | info.Timezone | America/Detroit |
So, now by declaring each method on the struct, when you run the code like so:
package main
import (
"fmt"
"log"
"net"
"github.com/ipinfo/go/v2/ipinfo"
)
func main() {
const token = "$YOUR_TOKEN"
client := ipinfo.NewClient(nil, nil, token)
const ip_address = "8.8.8.8"
info, err := client.GetIPInfo(net.ParseIP(ip_address))
if err != nil {
log.Fatal(err)
}
fmt.Printf("IP : %v\\n", info.IP)
fmt.Printf("ASN & Organization : %v\\n", info.Org)
fmt.Printf("City: %v\\n", info.City)
fmt.Printf("Region: %v\\n", info.Region)
fmt.Printf("Country: %v\\n", info.Country)
fmt.Printf("Country Name: %v\\n", info.CountryName)
fmt.Printf("European IP Address: %v\\n", info.IsEU)
fmt.Printf("Location (Latitude, Longitude): %v\\n", info.Location)
fmt.Printf("Postal Code: %v\\n", info.Postal)
fmt.Printf("Timezone: %v\\n", info.Timezone)
fmt.Printf("Hostname: %v\\n", info.Hostname)
fmt.Printf("Bogon IP Address: %v\\n", info.Bogon)
fmt.Printf("Anycast IP Address: %v", info.Anycast)
}
You will get an output like this:
IP : 8.8.8.8
ASN & Organization : AS15169 Google LLC
City: Mountain View
Region: California
Country: US
Country Name: United States
European IP Address: false
Location (Latitude, Longitude): 37.4056,-122.0775
Postal Code: 94043
Timezone: America/Los_Angeles
Hostname: dns.google
Bogon IP Address: false
Anycast IP Address: true
Outputting to JSON
If you want, you can output the entire response to JSON test using the json.Marshal
command. For that, you need to import the encoding/json
library.
package main
import (
"encoding/json" // this is required
"fmt"
"log"
"net"
"github.com/ipinfo/go/v2/ipinfo"
)
func main() {
const token = "YOUR_TOKEN"
client := ipinfo.NewClient(nil, nil, token)
const ip_address = "8.8.8.8"
info, err := client.GetIPInfo(net.ParseIP(ip_address))
if err != nil {
log.Fatal(err)
}
// marshalling to JSON
jsonified_content, _ := json.Marshal(info)
fmt.Println(string(jsonified_content))
}
In this case, your output would look something like this:
{
"ip": "8.8.8.8",
"hostname": "dns.google",
"anycast": true,
"city": "Mountain View",
"region": "California",
"country": "US",
"country_name": "United States",
"loc": "37.4056,-122.0775",
"org": "AS15169 Google LLC",
"postal": "94043",
"timezone": "America/Los_Angeles"
}
Getting more from your Go/IPinfo Package
That is the basics of the IPinfo IP Lookup. The client
handler has a bunch of methods that can be quite helpful, such as:
GetIPMap
⇒ Create an interactive map from IP addressesGetBatch
⇒ Do batch lookup or bulk lookup of IP addresses, CIDR, ASN, Netblocks etc.GetASNDetails
⇒ Lookup ASN and company information
On the paid tiers, you can get more information such as:
- Privacy (VPN/Proxy) Detection
- ASN/Company Lookup
- Mobile Carrier Data
- Hosted Domains Data etc.
You can find more information from here:
- GitHub Repo: https://github.com/ipinfo/go
- Go Package Repository: https://pkg.go.dev/github.com/ipinfo/go/v2
- IPinfo Documentation: https://ipinfo.io/developers/
Check out our other how-to guides to get started with IPinfo’s IP insights and information. If you would like to know more about IPinfo and learn cool tips, tricks and hacks, follow us on Twitter or LinkedIn.