In this article, we will look into how to get started with getting IP address information (including IP geolocation) using the IPinfo C# / .NET SDK in a C# project. Whether it be a web application development using the ASP.NET framework, developing a backend service using the C# language or even in-game development using Unity, IPinfo’s IP address data is essential in IP data enrichment, cybersecurity, and fraud prevention.
Installing the IPinfo Library
If you want to integrate IPinfo’s API service and data in your .NET application using the C# language, you should use the IPinfo C# / .NET SDK. You can use the IPinfo API service using the HTTP client library. However, our official open-source SDK makes using our data more simplified and it comes with some additional helpful features.
You can find detailed instructions on using our SDK from it’s GitHub page. To install the SDK, you can choose any of these options:
Option 1: Install using Package Manager
Install-Package IPinfo
Option 2: Install using the dotnet CLI
dotnet add package IPinfo
Option 3: Install with NuGet
nuget install IPinfo
Once installed, you are ready to start using the package.
Getting Started with IPinfo library
After you have installed the IPinfo package, you can start by initializing a new dotnet console. This project is created using the following command.:
mkdir ipinfo_tutorial
cd ipinfo_tutorial
dotnet new console
dotnet add package IPinfo
This resulted in the Program.cs
and ipinfo_tutorial.csproj
files. We will modify the Program.cs
file.
Now that you have your project startup, follow through this starter code:
//namespace
using IPinfo;
using IPinfo.Models;
namespace IPinfoApp {
// Class declaration
class IPlookup {
// Main Method
static async Task Main(string[] args) {
////////////////////////////////
// initializing IPinfo client //
////////////////////////////////
// Get your IPinfo access token from: ipinfo.io/account/token
string token = "YOUR_TOKEN";
IPinfoClient client = new IPinfoClient.Builder()
.AccessToken(token)
.Build();
// making the API call
string ip = "170.206.134.144"; // IP address
IPResponse ipResponse = await client.IPApi.GetDetailsAsync(ip);
/////////////////////
// IPinfo Insights //
/////////////////////
Console.WriteLine($"IP address: {ipResponse.IP}");
Console.WriteLine($"City: {ipResponse.City}");
Console.WriteLine($"Region / State: {ipResponse.Region}");
Console.WriteLine($"Country : {ipResponse.Postal}");
Console.WriteLine($"Country: {ipResponse.Country}");
Console.WriteLine($"Country Name: {ipResponse.CountryName}");
Console.WriteLine($"Geographic Coordinate: {ipResponse.Loc}");
Console.WriteLine($"Contitnent: {ipResponse.Continent.Name}");
Console.WriteLine($"Is EU?: {ipResponse.IsEu}");
}
}
}
Let’s break down this starter code.
Firstly, we are declaring the namespaces for the IPinfo packages we installed with:
// namespace
using IPinfo;
using IPinfo.Models;
Then we are initializing the IPinfo client. For this section, you should have your IPinfo access token. Signup to IPinfo for a free account and get your access token from the account dashboard. Then replace the YOUR_TOKEN
placeholder with your actual access token.
// Get your IPinfo access token from: ipinfo.io/account/token
string token = "YOUR_TOKEN";
IPinfoClient client = new IPinfoClient.Builder()
.AccessToken(token)
.Build();
And finally, we are retrieving IP address data, which in this case is IP to Geolocation information. Here we are using the asynchronous API method via GetDetailsAsync
. You can also use the synchronous method, GetDetails
as well.
For this lookup, we are only accessing the IP geolocation API response (city, region, country, etc.) and some package-specific features (continent, IsEU, etc.)
// making the API call
string ip = "170.206.134.144"; // IP address
IPResponse ipResponse = await client.IPApi.GetDetailsAsync(ip);
/////////////////////
// IPinfo Insights //
/////////////////////
Console.WriteLine($"IP address: {ipResponse.IP}");
Console.WriteLine($"City: {ipResponse.City}");
Console.WriteLine($"Region / State: {ipResponse.Region}");
Console.WriteLine($"Country : {ipResponse.Postal}");
Console.WriteLine($"Country: {ipResponse.Country}");
Console.WriteLine($"Country Name: {ipResponse.CountryName}");
Console.WriteLine($"Geographic Coordinate: {ipResponse.Loc}");
Console.WriteLine($"Contitnent: {ipResponse.Continent.Name}");
Console.WriteLine($"Is EU: {ipResponse.IsEu}");
If you would like to get other information, check out the method reference table below.
Now that we have walked through the standard IPinfo IP lookup code, we can run the program using the dotnet run
command from the terminal. After the program finishes running, we get the following result:
IP address: 170.206.134.144
City: Bellevue
Region / State: Washington
Country : 98006
Country: US
Country Name: United States
Geographic Coordinate: 47.5614,-122.1552
Contitnent: North America
Is EU: false
Getting more out of the IPinfo library
Depending on the pricing tier you are in, you can have access to different kinds of IP address information.
IP Lookup Response | Fields | |
---|---|---|
General Fields | ipResponse |
IP, City, Country, CountryName, Hostname, Loc, Latitude, Longitude, Org, Postal, Region, Timezone, Anycast, Bogon, IsEU, CountryFlag, CountryCurrency |
ASN | ipResponse.Asn |
Asn, Domain, Name, Route, Type |
IP to Company | ipResponse.Company |
Domain, Name, Type |
IP to Mobile Carrier | ipResponse.Carrier |
Name, Mnc, Mcc |
IP to Privacy Detection | ipResponse.Privacy |
Hosting, Proxy, Relay, Tor, Vpn, Service |
IP to Abuse Contact | ipResponse.Abuse |
Address, Country, Email, Name, Network, Phone |
Hosted Domains | ipResponse.Domains |
Domains, Total |
You can check out our knowledge base articles to learn more:
- [Privacy Detection] Get privacy, proxy and VPN detection information from an IP address using the C# language.
- [ASN & Company Database] Lookup ASN and Company information from an IP address using the C# programming language.
- [IP to Mobile Carrier] Get mobile carrier information from a phone’s IP address using the C# language
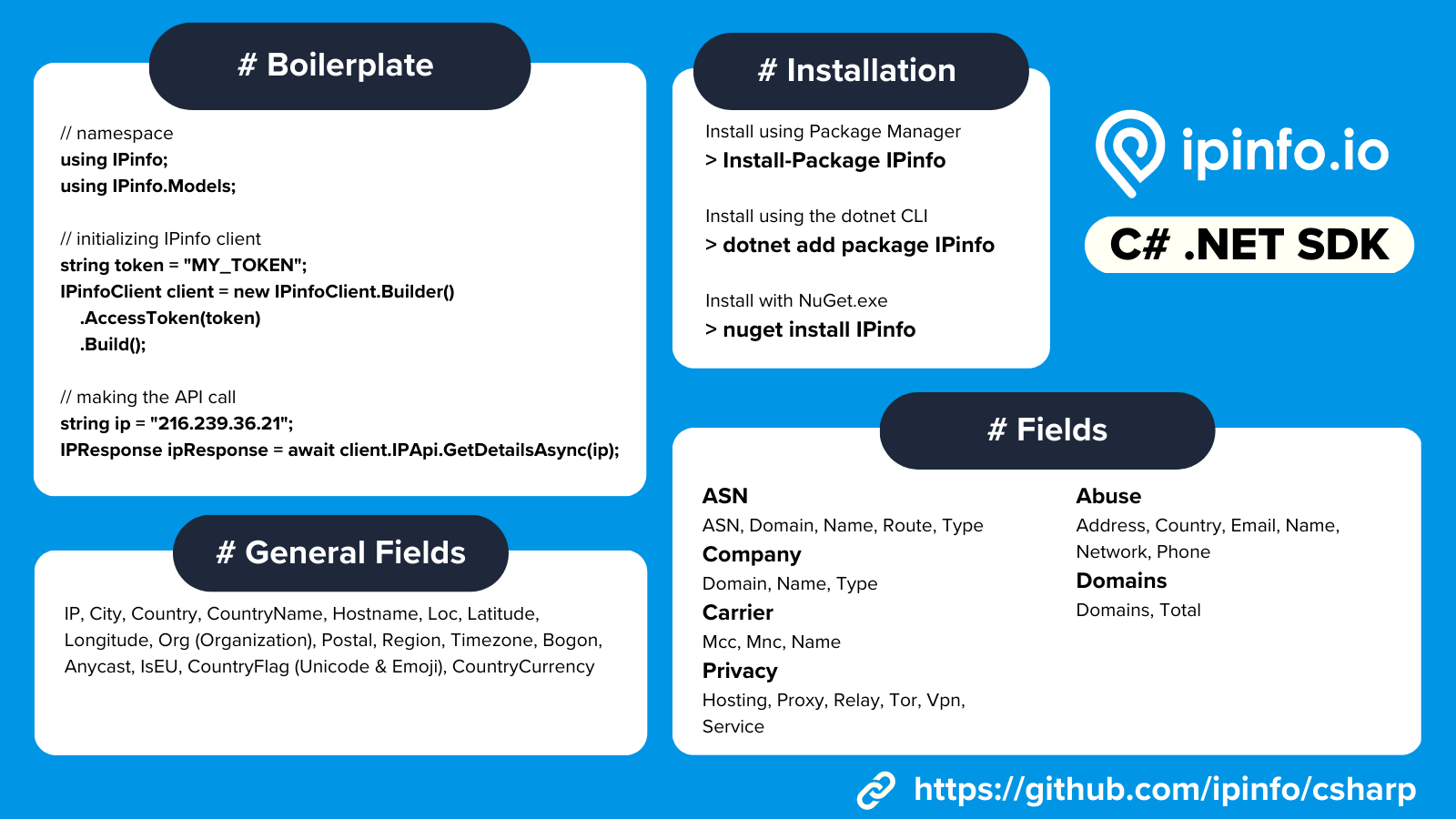
Getting Started with IPinfo Databases
Aside from our API, we also provide IP data through our database products. We deliver our IPinfo database in 3 different formats: CSV, JSON and MMDB. You can check out this article to learn more: How to choose the best file format for your IPinfo database?
In utilizing our database product in a C# program, you will most likely be looking up IP address data using the MMDB database. In that case, you should use MaxMind’s DB reader package.
To get started, install the MaxMind.Db
package:
dotnet add package MaxMind.db
We will be using our IPinfo IP to Location database for our MMDB database and will be looking up an IPv6 IP address: 2001:428:7003:8000::
Like before, let’s start with the basic starter code:
using MaxMind.Db; // mmdb reader library
using System.Net; // used to parse IP address input
namespace IPinfoMMDB {
public class Program {
private static void Main(string[] args) {
// creating the reader object and providing the path to the mmdb file
using(var reader = new Reader("location.mmdb")) {
// input IP address
var ip = IPAddress.Parse("2001:428:7003:8000::");
// looking up the IP address and outputting the result to dictionary
var ipData = reader.Find < Dictionary < string,
string >> (ip);
// if there is a match printing out the result
if (ipData is not null) {
foreach(var val in ipData) {
Console.WriteLine($"{val.Key}: {val.Value}");
}
}
}
}
}
}
From this, the output is:
city: Monroe
country: US
geoname_id: 4333669
lat: 32.5286
lng: -92.1061
postal_code: 71201
region: Louisiana
region_code: LA
timezone: America/Chicago
So, let’s break it down. First, you need to import the necessary packages: MaxMind.Db
, which will be used to read the MMDB file, and System.Net
, which will be used to parse the input IP address and will support both IPv4 and IPv6 IP addresses.
using MaxMind.Db;
using System.Net;
The core operation involves reading the MMDB file and creating the reader
object. Then we look up the IP address using the reader
object and output the result to the ipData
dictionary. If we have successfully found the data, we can print out its content using a foreach
loop. If the data is not available, we will get a null response.
{
using (var reader = new Reader("location.mmdb"))
{
var ip = IPAddress.Parse("2001:428:7003:8000::");
var ipData = reader.Find<Dictionary<string, string>>(ip);
if (ipData is not null){
foreach (var val in ipData)
{
Console.WriteLine($"{val.Key}: {val.Value}");
}
}
}
}
Because we are outputting the lookup content in a dictionary format, we can also access each field individually values by their Key.
var ipData = reader.Find<Dictionary<string, string>>(ip);
if (ipData is not null){
Console.WriteLine($"City: {ipData["city"]}");
Console.WriteLine($"Country: {ipData["country"]}");
Console.WriteLine($"Latitude: {ipData["lat"]}");
Console.WriteLine($"Longitude: {ipData["lng"]}");
Console.WriteLine($"Postal Code: {ipData["postal_code"]}");
Console.WriteLine($"Region: {ipData["region"]}");
Console.WriteLine($"Region Code: {ipData["region_code"]}");
Console.WriteLine($"Geoname ID: {ipData["geoname_id"]}");
Console.WriteLine($"Timezone: {ipData["timezone"]}");
}
If you would like to know about IPinfo’s database and its schemas, check out our documentation page.
One thing to note is that you should not create the reader
object every time you query a single IP address, since this is a computationally expensive process. You should be aware of proper caching methods as well. If you are looking to query individual IP addresses outside the C# / .NET environment, we highly recommend checking out our mmdbctl utility.
Now that you are familiar with using our API and databases in .NET framework, feel free to check some of our other how-to guides. If you want to level up and get more insights such as IP to privacy detection, IP company and other information free feel to check out our offerings.
For more IP data tips from other developers, follow us on Twitter or LinkedIn.